Instructions/Lectures
- Introduction: Problem and problem solving,
Algorithms, complexity analysis etc.
- How to write algorithms: psedocodes (using
elementary addition algorithm)
- Sorting algorithms
- Complexity Analysis of Insertion Sort.
- Asymptotic Behavior of Algorithms
- Asymptotic Efficiency of Algorithms: Formal and
mathematical definitions and properties.
- Methods for Asymptotic Analysis: Tight bound,
upper bound, lower bound etc.
- Comments on growth functions, bounding
summations, recurrences, graphs, trees, etc.
- Dynamic Programming Technique.
- Basic Elements of Dynamic Programming.
- Applicability of Dynamic Programming.
- Chain Matrix Multiplication Problem
- Naive Algorithm the Problem
- Dynamic Programming Approach for the Problem
- Dynamic Programming Formulation for the
Problem.
- Implementation of Dynamic Programming Rules
- Example: Chain Matrix Multiplication
- Recursive Implementation of the Problem
- Memoization
- Introduction: Polygons and Triangulations
- Minimum-Weight Convex Polygon Triangulation
- Correspondence to Binary Trees
- Dynamic Programming Solution for Triangulation
- Convex Hull Algorithms
- Convex Hull Problem and Approaches
- Determination of Extreme Edges
- Graham Scan Algorithm
- Running Time Computations
- Amortized Analysis: Aggregate Method and Stack
Operations (multipop)
- Correctness of Graham Scan
- Comments on Problem Reduction
- Reduction Example: Use of Hull Algorithm to
Sort.
- Conclusion: Some more comments on problem and
problem solving, Comments on inductive reasoning, problem or
statement formulation, two working examples.
Our Main Source:
- Thomas Cormen,
Charles Leiserson, Ronald Rivest, and
Cliff
Stein, Introduction to Algorithms, McGraw Hill Publishing Company and MIT Press, 2001 (2nd Edition).
|
 Have a Great Summer! 
|
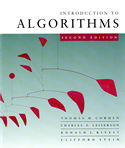 |